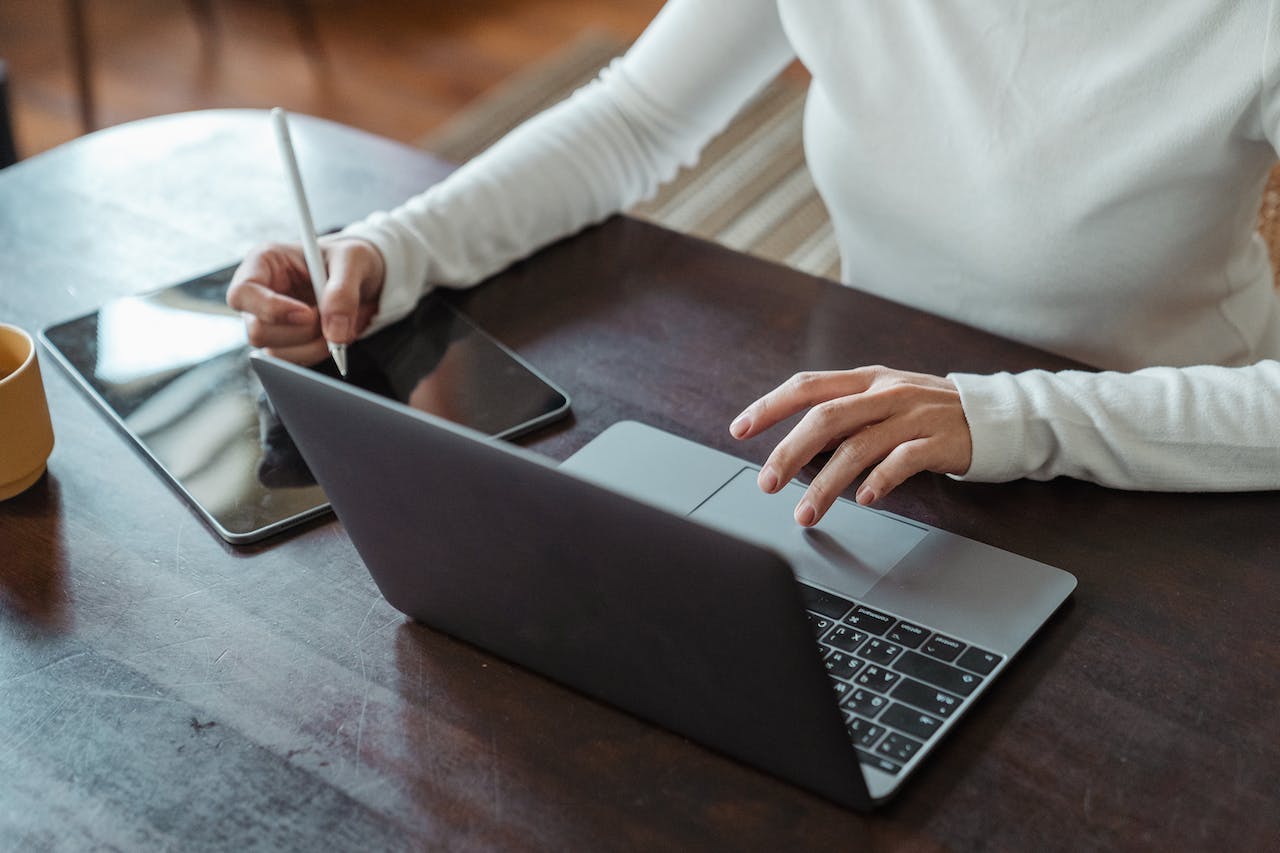
Multiprocessing and Multithreading in Python
Abir Jameel
- January 9, 2024
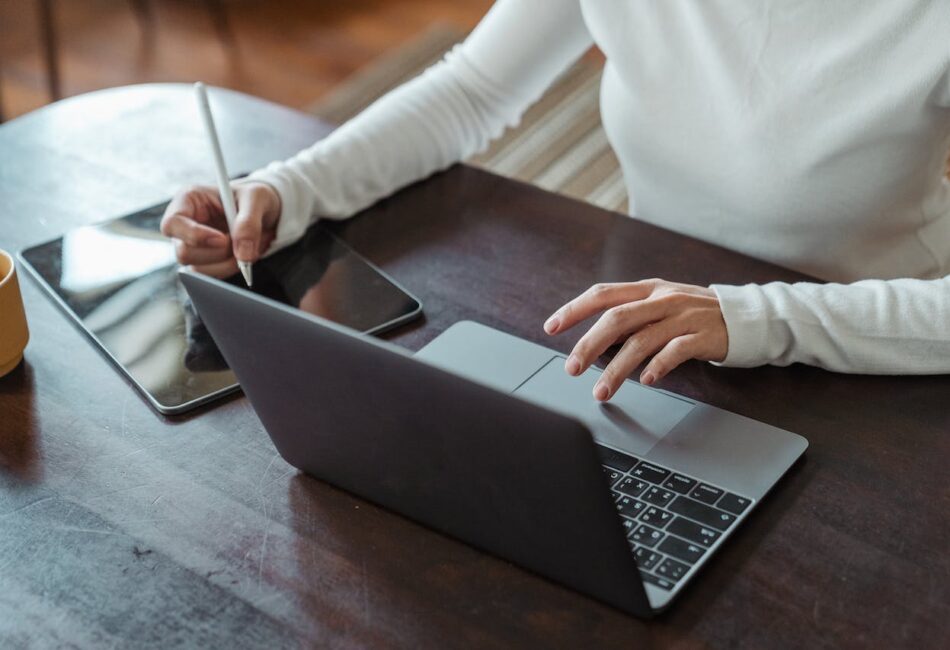
Introduction
We all know how Python is famous among data scientists and people doing scientific computations, here is a new lesson to learn about multithreading in Python, we will answer simple questions like what is multithreading? How can you use it in your programs, and what benefit you are going to get? Will it speed up your program? Well, Let’s go through the sections below to get the answers.
Understand Multiprocessing and Multithreading
To understand multithreading first, we need to understand what a process is. What a thread is. A program in execution in a separate address space is a process, each process has its own Python interpreter and memory space, and it runs independently of other processes.
Now let’s look at the thread, a thread is the smallest unit of execution within a process. A process can have multiple threads and each thread runs concurrently. The ‘threading’ module in Python provides a way to create and manage threads.
As it should be very intuitive now to understand what is multithreading and multiprocessing, we will take each one by one.
Multiprocessing is a technique where multiple processes run across multiple processors or cores simultaneously. The ‘multiprocessing’ module in Python is commonly used to create and manage processes, It allows you to parallelize your program in a true sense taking advantage of multicore processors and improving performance by providing each process a Python Interpreter.
Multithreading on the other hand creates multiple threads simultaneously, In Python multithreading threads can run only concurrently because of Global Interpreter Lock or GIL which does not allow parallel execution of threads.
How to use Multithreading in Python
If you want to break your program into tasks and subtasks and then execute them simultaneously then multithreading is the best way. To understand more how we take up an example –
As we already know we can use `threading` module
import threading
def worker_function():
print("Worker thread")
if __name__ == "__main__":
# Create a thread
my_thread = threading.Thread(target=worker_function)
# Start the thread
my_thread.start()
# Wait for the thread to finish
my_thread.join()
print("Main thread")
In this example, a new thread is created using the `threading.Thread` class, and the `target` parameter specifies the function to be executed in the new thread. The `start()` method initiates the execution of the thread and the `join()` method is used for the thread to finish before moving on to the main thread. Python threads are more suitable for I/O-bound tasks e.g. network operations or file I/O than for CPU-bound tasks.
About Author
Abir Jameel
Recent Comments
Categories
Archives
- May 2024 (1)
- January 2024 (1)
- October 2023 (1)
- July 2022 (1)
- October 2021 (2)
Categories
- Advanced 1
- Beginner 2
- Intermediate 2
- Tech 2
